Contents
Introduction to React
React is a popular open-source JavaScript library used for building interfaces (UIs) and front-end applications. It was developed by Facebook and released in 2013. React focuses on creating reusable UI components that manage their own state and can be efficiently updated when data changes. This approach to building UIs is often referred to as “declarative” programming.
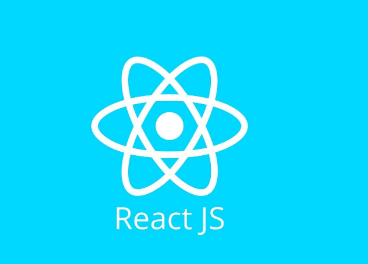
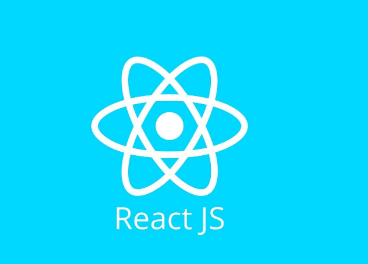
What is React?
React is an open-source JavaScript library that is used for building interfaces (UIs) and interface components. It was developed by Facebook and is maintained by Facebook along with a community of developers and companies. React is widely used in modern web development to create dynamic and interactive UIs for web applications.
At its core, React provides a set of tools and abstractions that simplify the process of creating UI components and managing their state. Here are some key aspects of React:
Component-Based Architecture:
React encourages developers to break down UIs into smaller, reusable components. Each component represents a self-contained piece of the interface, which makes it easier to manage and maintain complex UIs.
Virtual DOM:
Reat introduces a concept called the Virtual DOM. Instead of directly manipulating the browser’s DOM, React creates a virtual representation of the DOM in memory. When data changes, React calculates the difference between the current virtual DOM and the new one, and then updates the actual DOM with only the necessary changes. This process improves performance by minimizing the number of actual DOM manipulations.
Reactive Updates: React efficiently updates the interface when the underlying data changes. When data within a component’s state changes, React re-renders only the affected components and their children, ensuring that the UI remains consistent with the data.
Key concepts and features of React include:
Components:
Components are the building blocks of a React application.
They represent a piece of the UI and can be as simple as a button or as complex as a whole page.
Components can be divided into two main types:
Functional components and class components.
Functional components
are simpler and use JavaScript functions to define the UI.
class components are defined using ES6 classes and have additional features like managing state and lifecycle methods.
Virtual DOM:
One of React’s most significant innovations is the Virtual DOM. Instead of directly updating the actual DOM (the browser’s representation of the web page), React creates a virtual representation of the DOM in memory. When data changes, React calculates the difference between the current virtual DOM and the new one and updates the real DOM efficiently, reducing the number of actual changes to the browser’s rendering.
Declarative Syntax:
With React, developers describe what the UI should look like based on the current state, and React takes care of updating the actual DOM to match that description. This approach is in contrast to the traditional imperative approach, where developers manually manipulate the DOM to achieve the desired UI changes.
This is in contrast to the imperative approach where you would directly manipulate the DOM to make changes.
State Management:
React allows components to have their own local state, which represents data that can change over time. This local state is managed within the component and can be updated using the setState method. This mechanism helps in creating interactive and dynamic UIs.
- React components can have their own local state, which represents data that can change over time.
- State is used to manage dynamic content and When the state of a component changes, React automatically updates the UI to reflect those changes.
Props (Properties):
- Props are a way to pass data from a parent component to a child component.
- They are read-only and help in creating reusable and configurable components.
- Props allow you to customize the behavior and appearance of components.
Component Lifecycle:
Class components in React have a set of lifecycle methods that allow you to perform actions at different stages of a component’s existence, such as when it’s created, updated, or unmounted from the DOM.
These methods provide hooks for performing tasks like data fetching, setting up timers, and cleaning up resources.
React Hooks:
Introduced in React 16.8, hooks are functions that allow developers to use state and other React features in functional components without needing to write class components. Hooks enable functional components to have the same capabilities as class components, simplifying the code and promoting code reuse.
Hooks provide a more concise and flexible way to manage state and side effects.
React Router:
While React is primarily focused on UI components, React Router is a library used for handling navigation and routing within a single-page application (SPA). It allows developers to create multi-page-like experiences in SPAs.
- React Router is a popular library used for handling navigation and routing in React applications.
- It enables you to create single-page applications (SPAs) with multiple views or pages.
Context API:
The Context API allows you to share state and data between components without having to pass props through multiple layers of components.
It’s particularly useful for managing global state in larger applications.
React has a vast ecosystem of tools, libraries, and community support, making it a powerful choice for building modern web applications.
It’s commonly used alongside other technologies such as Redux (for advanced state management), Webpack (for bundling assets), and Babel (for transpiling modern JavaScript code).
Whether you’re building a simple interface or a complex web application, React’s modular and efficient design can help you create responsive and interactive experiences for your s.
Here are some additional important concepts and best practices when working with React:
Conditional Rendering:
React allows you to conditionally render components or elements based on certain conditions. This is often achieved using JavaScript expressions within JSX (JavaScript XML) to determine whether a component should be displayed or not.
Lists and Keys:
When rendering lists of elements in React, each element should have a unique “key” prop. This helps React efficiently update and re-render the list when items are added, removed, or reordered.
Forms:
Handling form input and interactions is a common task in web applications. React provides a way to manage form inputs and their state, and it encourages using controlled components, where the form input’s value is controlled by React state.
Styling:
React doesn’t prescribe a specific way to style components. You can use traditional CSS, CSS-in-JS libraries, or CSS modules to style your components. Popular libraries for styling React components include styled-components, Emotion, and CSS Modules.
Server-side Rendering (SSR) and Static Site Generation (SSG):
React can be used for server-side rendering, where the initial HTML is generated on the server before being sent to the client. This can improve initial page load times and SEO. Additionally, React can be used for static site generation, where pre-rendered HTML files are generated at build time for better performance and security.
Error Handling:
React components can implement error boundaries to catch and handle errors that occur during rendering. This prevents the entire UI from crashing due to a single component’s error.
Performance Optimization:
While React’s Virtual DOM helps optimize rendering performance, you can further optimize your application by using techniques like memoization (via the React.memo higher-order component) to prevent unnecessary re-renders of components.
Component Composition:
React encourages breaking UIs into smaller, reusable components. These components can be composed together to build complex interfaces. This promotes maintainability and reusability.
Debugging:
React provides tools and browser extensions like React DevTools that help you inspect the component hierarchy, state, and props, making it easier to debug your application.
Testing:
React applications can be tested using various testing libraries and frameworks like Jest and React Testing Library. Writing tests ensures the reliability and correctness of your components and application logic.
Lifecycle Changes (with React 17 and Beyond):
With the introduction of React 17, certain lifecycle methods like componentWillUnmount have been deprecated. React’s focus is shifting towards function components and hooks, so developers are encouraged to use the useEffect hook for handling side effects and cleanup.
Type Safety:
To enhance code quality and prevent runtime errors, you can use TypeScript or Flow to add static type checking to your React code. This helps catch type-related issues during development.
Remember that React is continuously evolving, and staying up-to-date with the latest best practices, tools, and libraries is essential for building robust and maintainable applications. The official React documentation, online tutorials, and the React community are excellent resources for deepening your understanding and skills in React development.
Importance of React
React holds significant importance in modern web development for several reasons:
- Efficient UI Updates: React’s Virtual DOM and reconciliation algorithm enable efficient updates to the Instead of re-rendering the entire DOM, React calculates and applies only the necessary changes, resulting in better performance and smoother experiences.
- Component-Based Architecture: React’s component-based architecture promotes modular development. Components can be reused, composed, and tested independently, leading to more maintainable and scalable codebases.
- Declarative Syntax: React’s declarative approach allows developers to focus on describing what the UI should look like based on the data and state, rather than worrying about how to update the DOM. This leads to more readable and predictable code.
- Reusability: With React, you can create a library of reusable components, saving development time and effort. These components can be shared across projects or with the community, fostering a culture of collaboration.
- State Management: React simplifies state management within components. The ability to manage local state, along with tools like the Context API and third-party libraries like Redux, makes it easier to handle complex data flows and
- Rich Ecosystem: React has a vast ecosystem of tools, libraries, and resources built around it. This includes routing libraries, styling solutions, state management options, testing frameworks, and more. This ecosystem accelerates development and provides solutions to common challenges.
- Large Community: React has a massive and active community of developers, which means there are abundant tutorials, documentation, and open-source projects available. This community support helps developers learn, troubleshoot, and share knowledge effectively.
- Performance: React’s focus on efficient rendering, along with optimizations like lazy loading and code splitting, contributes to better application performance. This is crucial for delivering fast and responsive
- SEO and Server-Side Rendering (SSR): React’s server-side rendering capabilities allow search engines to index content more effectively. This is essential for improving search engine optimization and ensuring that content is accessible to s.
- Compatibility and Adaptability: React can be integrated into existing projects, coexisting with other libraries and frameworks. Additionally, it can be used for various platforms, including web, mobile (React Native), and desktop (Electron), making it versatile for different development scenarios.
- Learning Curve: React’s core concepts are relatively straightforward to understand, especially for developers familiar with JavaScript. This accessibility makes it easier for newcomers to the world of front-end development to get started.
- Industry Adoption: React is widely adopted by companies of all sizes, from startups to tech giants. This means that learning and using React increases your employability and job opportunities.
In summary, React’s emphasis on efficient rendering, component reusability, and its supportive ecosystem make it a powerful choice for building modern and -friendly web applications. Its influence extends beyond web development, with its usage in mobile app development and other areas, making it a skill with broad applicability in the software development landscape.
Uses of react
React is used across a wide range of applications and industries due to its versatility and efficiency in building interfaces. Here are some common uses of React:
Web Applications:
React is widely used to build single-page applications (SPAs) and dynamic web interfaces. It’s suitable for a variety of web applications, from simple landing pages to complex e-commerce platforms and social media sites.
Interfaces:
React’s component-based architecture and declarative syntax make it ideal for creating interactive and responsive interfaces. It’s used for building UI components like buttons, forms, menus, modals, and more.
Content Management Systems (CMS):
Many content management systems leverage React to provide a dynamic and engaging editing experience for s. React’s reactivity allows for real-time updates and previews as content is being edited.
E-commerce Platforms:
React is commonly used to build the front-end of e-commerce websites. Its ability to manage complex interfaces, product catalogs, and shopping carts makes it a suitable choice for these platforms.
Social Media Applications:
Platforms that require real-time updates, notifications, and interactions, such as social media sites and messaging apps, benefit from React’s efficient rendering and state management capabilities.
Data Dashboards:
React is well-suited for building data visualization and analytics dashboards. Its ability to render dynamic charts, graphs, and data tables allows developers to create informative and interactive dashboards.
Online Marketplaces:
Online marketplaces that connect buyers and sellers often require complex interfaces to display products, manage transactions, and facilitate interactions. React can handle these requirements effectively.
Real-Time Applications:
Applications that rely on real-time data updates, such as live sports scores, financial tickers, or collaborative tools, can benefit from React’s ability to efficiently update the UI in response to changing data.
Booking and Reservation Systems:
Platforms that handle reservations, bookings, and scheduling require interactive calendars, availability displays, and forms. React can provide the dynamic experience needed for such systems.
Enterprise Applications:
React is used for building internal business applications like project management tools, customer relationship management (CRM) systems, and employee portals. Its component reusability and maintainability are advantageous for such applications.
Educational Platforms:
Online learning platforms and e-learning applications utilize React to create engaging interfaces for courses, quizzes, and interactive content.
Media Streaming Platforms:
Platforms that stream videos, music, or other media benefit from React’s ability to handle dynamic content updates and interactions.
Mobile Applications (React Native):
React can be used to build mobile applications using React Native, a framework that allows developers to create native mobile apps for iOS and Android using a single codebase.
Desktop Applications (Electron):
With the help of Electron, React can be used to build cross-platform desktop applications, making it possible to create apps for Windows, macOS, and Linux using web technologies.
Prototyping and MVPs:
React’s fast development cycle and modular architecture make it a great choice for quickly prototyping new ideas or creating minimum viable products (MVPs) to test concepts.
Progressive Web Apps (PWAs):
React is often used to build progressive web apps that combine the best of web and mobile experiences. PWAs offer offline access, fast loading times, and native-like interactions, and React’s component-based approach is well-suited for creating these applications.
Interactive Maps and Geolocation:
React can be used with mapping libraries like Leaflet or Mapbox to create interactive maps and geolocation-based applications, such as location services, travel planners, and geospatial data visualization.
Augmented Reality (AR) and Virtual Reality (VR) Experiences:
When combined with libraries like A-Frame, React can be used to create immersive AR and VR experiences that run in web browsers, making it accessible to a broader audience.
Gaming Interfaces:
React’s ability to handle dynamic content and real-time updates makes it suitable for building interfaces for web-based games and gamified applications.
Healthcare Applications:
React can be used to develop healthcare applications like patient portals, telemedicine platforms, appointment scheduling systems, and medical record management tools.
Financial and Banking Applications:
React’s ability to handle complex UIs, data visualization, and interactive elements is beneficial for creating financial dashboards, investment platforms, and online banking interfaces.
Travel and Hospitality Platforms:
Booking platforms, travel itineraries, hotel reservation systems, and tour planning applications often utilize React to provide engaging experiences.
Job Portals and Recruitment Platforms:
React’s interactive forms and dynamic search functionalities are useful for building job search platforms, application management systems, and applicant tracking systems.
IoT (Internet of Things) Interfaces:
React can be used to build interfaces for IoT devices and control panels that allow s to interact with and manage smart devices.
Weather and Forecasting Applications:
Applications that provide weather forecasts, live weather data, and weather maps can utilize React to render dynamic and up-to-date information.
e-Government Services:
Government websites and applications can leverage React to create -friendly interfaces for services such as tax filing, document submission, and online permit applications.
Community and Social Platforms:
Online communities, forums, and social networking platforms can utilize React’s dynamic UI capabilities to provide engaging experiences and facilitate interactions.
Language Learning and Education:
React can be employed to build language learning apps, educational games, and interactive educational content for students of all ages.
Art and Creative Applications:
React can be used to create interactive art installations, creative coding projects, and applications that allow s to generate visual or auditory content.
Personal Portfolio and Blog Websites:
Even for personal websites, React can be used to showcase your skills, projects, and blog posts with dynamic and visually appealing components.
React’s versatility and scalability make it suitable for a wide array of applications and industries. Its ability to create engaging experiences, coupled with its active community and extensive resources, makes it a powerful tool for modern software development.
History of react
React’s history is marked by significant developments and milestones that have shaped its growth and popularity. Here’s an overview of the key events in the history of React:
- 2011: Facebook engineer Jordan Walke starts working on a JavaScript library called “Fax.js,” which aimed to address performance issues in Facebook’s web app. This project eventually evolved into what we now know as React.
- 2013: React is officially released to the public in May. Its primary purpose is to simplify the process of building complex interfaces by introducing a component-based architecture and a virtual DOM. This initial version of React laid the foundation for its future growth.
- 2014: React gains traction within the developer community, but there’s still a learning curve and some resistance due to its new concepts. React team releases the Flux architecture pattern as a way to manage state in applications. However, Flux proves to be complex, leading to the development of other state management solutions like Redux.
- 2015: React Native is introduced by Facebook in March. It’s a framework for building mobile applications using React’s component-based model. Developers can create native iOS and Android apps using the same codebase, making cross-platform development more efficient.
- 2016: React continues to evolve, and more companies and projects adopt it. The React team introduces the concept of “fiber,” an internal reimplementation of the reconciliation algorithm that improves performance and paves the way for concurrent rendering.
- 2017: React 16 is released in September. This version introduces significant changes, including the Fiber-based reconciliation algorithm (still opt-in) and the introduction of “React Fragments” (nowadays known as “React Fragments”) for better component rendering. React 16 also brings support for error boundaries, which improve the stability of applications.
- 2018: The React team introduces React Hooks in React 16.8. Hooks are a set of functions that allow developers to use state and lifecycle features in functional components, eliminating the need for class components in many cases. This simplifies and enhances code reuse and composability.
- 2019: Concurrent Mode is introduced as an experimental feature, allowing React applications to perform better, particularly in scenarios involving complex UI updates and responsiveness. Concurrent Mode aims to make applications more efficient by breaking down large updates into smaller, manageable chunks.
- 2020: Concurrent Mode is still being developed, and the React team focuses on refining its implementation. The team also emphasizes server-side rendering (SSR) and improvements in streaming SSR to make applications load and become interactive more quickly.
- 2021: React 18 is anticipated as the next major release, focusing on Concurrent Mode and other performance optimizations. The React team continues to work on making Concurrent Mode more accessible and providing better tools for gradual adoption.
Throughout its history, React has gained widespread adoption and has become a cornerstone of modern web and application development. Its evolution has been driven by the goal of providing developers with efficient tools to create fast, responsive, and maintainable interfaces. The React team’s commitment to addressing developer pain points and continuously improving the library has contributed to its ongoing popularity.
React VS Javascript
It seems there might be a bit of confusion in your question. React and JavaScript are not directly comparable because they serve different purposes. React is a JavaScript library for building interfaces, while JavaScript is a programming language used for web development and other software applications. Let’s clarify the distinction:
JavaScript:
- JavaScript is a versatile, high-level programming language used primarily for web development.
- It’s an essential technology for creating interactive and dynamic websites, web applications, and even server-side applications using technologies like Node.js.
- JavaScript runs in web browsers and allows you to manipulate the Document Object Model (DOM), handle interactions, perform asynchronous operations, and much more.
- JavaScript can be used for a wide range of tasks, from simple client-side scripting to complex server-side logic.
React:
- React is a JavaScript library specifically designed for building
- It provides a declarative way to create reusable UI components and manage the state of a web application.
- React uses a virtual DOM and efficient updates to improve rendering performance and
- React is commonly used in conjunction with JavaScript to build interactive and dynamic web applications with complex
- In summary, React is a tool that is written in JavaScript and used to simplify the process of building interfaces, while JavaScript is the programming language that enables web development across a wide array of tasks and applications. They are not directly comparable but are often used together, with React being a framework that leverages JavaScript’s capabilities to build efficient and interactive
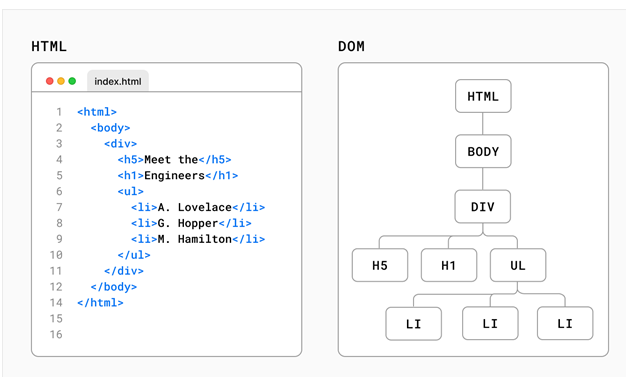
How does React Work?
React works by using a combination of its core principles, such as the Virtual DOM, component-based architecture, and declarative syntax, to efficiently update and render interfaces.
Here’s a step-by-step overview of how React works:
Component Creation:
Developers define UI components using React. Components can be functional components (using JavaScript functions) or class components (using JavaScript classes). These components are the building blocks of the interface.
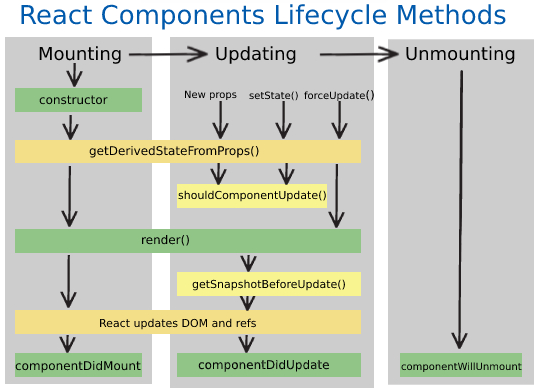
State and Props:
Components can have state, which represents data that can change over time. State is managed internally within the component and can be modified using the setState function.
Components can also receive data from their parent components through props (short for properties). Props are read-only and allow parent components to configure and pass data to their children.
Component Rendering:
When a component’s state or props change, React triggers a process called rendering. Rendering means creating a virtual representation of the UI component’s structure and content, often referred to as the “virtual tree” or Virtual DOM.
Virtual DOM Creation:
React creates a lightweight copy of the actual DOM, known as the Virtual DOM. This Virtual DOM is a tree structure that mirrors the structure of the actual DOM but is stored in memory rather than being part of the browser’s rendering.
Diffing and Reconciliation:
When a component’s state changes, React performs a process called “diffing” or “reconciliation.” It compares the previous Virtual DOM (before the state change) with the new Virtual DOM (after the state change) to identify the differences or updates needed to reflect the change.
Efficient Updates:
React’s diffing algorithm identifies the minimal number of changes required to update the Virtual DOM. This process optimizes performance by minimizing the actual updates that need to be made to the real DOM.
DOM Update:
After identifying the changes needed, React updates the real DOM efficiently by applying only the necessary changes. This process is often referred to as “reconciliation” or “re-rendering.”
Component Lifecycle and Hooks:
During the rendering and updating process, React triggers specific lifecycle methods or hooks (if using functional components with hooks). These methods allow developers to perform additional tasks, such as fetching data, managing subscriptions, or handling side effects.
Interactions and State Changes:
interactions (such as clicks or input) can trigger changes to a component’s state or props. When this happens, React follows the same process of rendering, diffing, and updating to ensure the interface reflects the new data.
Reusability and Composition:
React’s component-based architecture allows developers to compose complex UIs by combining and nesting smaller, reusable components. This approach promotes modularity and code reuse.
Overall, React’s approach of using a Virtual DOM and focusing on declarative updates allows developers to create efficient, responsive, and maintainable interfaces. By minimizing direct manipulation of the real DOM and optimizing updates, React enhances the performance and experience of web applications.
multichoice quiz with answers about this lesson
Sure, here’s a multiple-choice quiz to test your understanding of the concepts covered in the lesson about React. Each question has one correct answer and three incorrect options. After answering, I’ll provide the correct answers. Let’s get started:
1-What is React primarily used for?
- a) Creating server-side logic
- b) Building interfaces
- c) Managing backend databases
- d) Writing networking protocols
2-Which of the following is a core concept of React?
- a) Server-side rendering
- b) Imperative syntax
- c) Component-based architecture
- d) PHP scripting
3-What is the main advantage of React’s Virtual DOM?
- a) It directly manipulates the actual DOM
- b) It reduces the need for components
- c) It improves state management
- d) It optimizes DOM updates for better performance
4-What is JSX in React?
a) A JavaScript debugging tool
b) A Java-based syntax extension
c) A way to define styles in React
d) A syntax extension for embedding HTML-like code within JavaScript
5-Which React feature allows functional components to have state and lifecycle capabilities?
a) Functional composition
b) Component lifecycle
c) React Hooks
d) Virtual DOM
6-What’s the primary purpose of React Native?
a) Server-side scripting
b) Building native mobile apps
c) Creating backend services
d) Writing desktop applications
7-Which of the following is NOT a React lifecycle method?
a) componentDidMount
b) componentCreated
c) componentDidUpdate
d) componentWillUnmount
8-What’s the primary purpose of the React Router library?
a) Managing global application state
b) Handling navigation and routing in SPAs
c) Creating UI components
d) Optimizing DOM rendering
Answers:
- b) Building interfaces
- c) Component-based architecture
- d) It optimizes DOM updates for better performance
- d) A syntax extension for embedding HTML-like code within JavaScript
- c) React Hooks
- b) Building native mobile apps
- b) componentCreated
- b) Handling navigation and routing in SPAs
9-What does React’s declarative syntax mean?
a) It focuses on direct manipulation of the DOM
b) It describes how to change the DOM imperatively
c) It describes what the UI should look like based on the data
d) It simplifies the usage of AJAX requests
10-Which of the following is NOT a benefit of using React?
a) Improved performance with direct DOM manipulation
b) Reusability of components
c) Efficient updates with the Virtual DOM
d) Simplified state management
11-What is the purpose of React’s “props”?
a) To manage local state within a component
b) To define styles for a component
c) To pass data from parent to child components
d) To handle interactions
12-In React, what does the term “reconciliation” refer to?
a) The process of transforming JSX to JavaScript
b) The process of creating components using JSX
c) The process of calculating changes in the Virtual DOM and updating the actual DOM
d) The process of handling asynchronous tasks in React components
13-Which of the following is NOT a lifecycle method in React?
a) componentDidRender
b) componentDidUpdate
c) componentWillUnmount
d) componentWillMount
14-What is the primary advantage of using React Hooks over class components?
a) Better performance
b) Improved browser compatibility
c) Simpler management of component state and side effects
d) Enhanced support for CSS-in-JS libraries
Answers:
- c) It describes what the UI should look like based on the data
- a) Improved performance with direct DOM manipulation
- c) To pass data from parent to child components
- c) The process of calculating changes in the Virtual DOM and updating the actual DOM
- a) componentDidRender
- c) Simpler management of component state and side effects
References of this lesson
Here are some references you can explore to learn more about React:
React Official Documentation: The official documentation is a comprehensive resource for learning about React’s concepts, API, and best practices. You can find it at:
React Official Documentation
React Native Official Documentation: If you’re interested in React Native for mobile app development, the official documentation provides detailed information:
React Native Official Documentation
React Router Documentation: To learn about routing in React applications, the React Router documentation is a great place to start:
React Router Documentation
React Hooks Documentation: For a deep dive into React Hooks and how they work, you can explore the Hooks documentation:
React Hooks Documentation
React Patterns: A collection of design patterns, techniques, tips, and tricks for working with React:
React Patterns
Egghead.io: This platform offers a wide range of video tutorials on React and related technologies:
Egghead.io – React
Scrimba: Scrimba offers interactive coding tutorials, including React courses that allow you to code along with the instructor:
Scrimba – React
React Podcast: A podcast that covers topics related to React and the front-end development ecosystem:
React Podcast
Reactiflux: A community of React developers on Discord where you can discuss React-related topics and ask questions:
Reactiflux Discord
GitHub Repository: The source code for React and its related projects can be found on GitHub:
React GitHub Repository
These resources should provide you with a strong foundation to learn and master React. Remember that the technology landscape evolves, so it’s important to stay updated by exploring official documentation and community discussions.
كورسات عربي
كورسات انجلش
CoursesE
Curriculum
- 1 Section
- 1 Lesson
- 10 Weeks
- React JavaScript Tutorial1
hello there and thank you for your information – I have certainly
picked up anything new from right here. I did however expertise some technical
issues using this web site, as I experienced to reload
the website many times previous to I could get it to load correctly.
I had been wondering if your web hosting is OK? Not that
I am complaining, but sluggish loading instances times will very frequently
affect your placement in google and can damage your high quality score if ads and
marketing with Adwords. Well I am adding this RSS to my e-mail
and could look out for a lot more of your respective fascinating
content. Make sure you update this again soon..
Lista escape roomów
Very interesting points you have remarked, appreciate it for posting.?
Hello there! This is my first visit to your blog! We are a group of volunteers and starting a new initiative in a community in the same niche. Your blog provided us beneficial information to work on. You have done a extraordinary job!
Good day! I know this is kind of off topic but I was wondering which blog platform are you using for this site? I’m getting sick and tired of WordPress because I’ve had issues with hackers and I’m looking at options for another platform. I would be great if you could point me in the direction of a good platform.
I have observed that car insurance businesses know the cars which are susceptible to accidents along with risks. Additionally , they know what form of cars are susceptible to higher risk and the higher risk they’ve got the higher your premium rate. Understanding the easy basics of car insurance will help you choose the right style of insurance policy that will take care of the needs you have in case you happen to be involved in an accident. Thank you sharing your ideas for your blog.
It’s a shame you don’t have a donate button! I’d most certainly donate to this fantastic blog! I suppose for now i’ll settle for bookmarking and adding your RSS feed to my Google account. I look forward to fresh updates and will talk about this website with my Facebook group. Talk soon!
Heya! I’m at work surfing around your blog from my new iphone! Just wanted to say I love reading your blog and look forward to all your posts! Carry on the great work!
Thanks for some other informative site. Where else could I get that kind of info written in such an ideal manner? I have a venture that I am simply now running on, and I’ve been on the glance out for such info.
There are some interesting deadlines on this article but I don?t know if I see all of them middle to heart. There’s some validity but I’ll take hold opinion until I look into it further. Good article , thanks and we wish extra! Added to FeedBurner as properly
I like what you guys are up also. Such clever work and reporting! Keep up the superb works guys I?ve incorporated you guys to my blogroll. I think it will improve the value of my site 🙂
I don?t even know how I ended up here, but I believed this put up was great. I don’t know who you’re however definitely you’re going to a well-known blogger when you are not already 😉 Cheers!
I savor, cause I discovered exactly what I was taking a look for. You have ended my 4 day lengthy hunt! God Bless you man. Have a great day. Bye
The greatest aspect effect of steroid use is the physique stops naturally producing testosterone
since it’s getting greater than enough with the medication. So when someone decides to cease
taking steroids, they’ll expertise a “hormone crash”.
This crash will produce withdrawal signs similar to any drug abuse like melancholy, headaches, anxiousness, loss of urge for food, nausea and more.
Not solely this, however long run abuse of steroids might lead to extra critical situations like coronary heart and liver disease.
When a person stops taking steroids, that is where post cycle therapy steps in. When using exogenous testosterone, both within the type of testosterone replacement
therapy (TRT) or in an anabolic steroid cycle, it may be very important have
a proper post-cycle remedy (PCT) plan in place.
The function of PCT is to help the body restore its pure testosterone manufacturing and keep away from
any undesirable unwanted effects or hormonal imbalances.
This 6-week cycle offers a good stability between benefits and unwanted facet effects.
If you’re going to run a Testolone cycle, take a glance at my full Testolone (RAD-140) cycle guide.
A dose of 15mg is unlikely to cause testosterone suppression, but any dose can still put you at
unknown risks of different unwanted effects.
Dosage varies primarily based on What Is The Purpose Of Anabolic Steroids? your targets are and how skilled
and confident you are in utilizing steroids and controlling side effects.
Most bodybuilders will use round 500mg every week of testosterone cypionate for bulking functions.
Some will begin as little as 200mg or 300mg weekly, whereas hardcore bodybuilders
are known to extend right as a lot as 1000mg per week.
It has a protracted half-life, so you will get away with taking
your complete dose in just one injection each week.
Whether you want to seem like the Arnie of yesteryear or wish to get your body into
peak conditioning with essentially the most ripped physique you can muster, we know that
many individuals do it with an informed use of steroids.
Because it aids in reestablishing normal hormone ranges and
guards in opposition to the negative penalties of excessive prolactin levels, cabergoline can additionally be suggested for post-cycle treatment (PCT).
Two weeks following the conclusion of the cycle, cabergoline ought to be begun and maintained for 4-6 weeks.
Additionally, you must take a sabbatical
for at least one month between every cycle. Researchers checked
out how cabergoline affected the levels of
development hormone in healthy people. According to the
research, cabergoline can increase growth hormone levels by as a lot as a two-fold.
Tren will deliver super lean muscle and energy gains
without water retention and boosting nutrient effectivity.
Deciding to use anabolic steroids for the primary time is an enormous step.
After all, you’re thinking of modifying your body’s chemistry and pure
processes, and that’s not something to take lightly.
You can take your physique and efficiency to a stage you’d never imagined.
The TRT cruise in between cycles goes to take care of your testosterone function.
Most users discover it simple to maintain features when blasting and
cruising, but it’s a long-term dedication. Using anti-progestogenic
ancillaries on a Nandrolone cycle will defend in opposition to these antagonistic results, particularly gynecomastia and sexual dysfunction (see below).
You might assume you’re feeling fine, however you will have no means of figuring out what
effects SARMs have over the long run. To perceive the potential advantages that SARMs can have for ladies, it’s essential
to have a primary understanding of what an SARM is and why it’s totally different from an anabolic steroid.
It’s important to understand that all SARMs are classed as investigational medication only,
with none yet being permitted for any use.
Cardarine at 20mg every day and Ostarine at 20mg daily is a common technique.
Nonetheless, many individuals will discover that a decrease dose provides substantial benefits while reducing unwanted
aspect impact dangers. You’ll get a boost to your metabolism thanks to Cardarine, which hastens fats burning,
and you’ll notice increased power and endurance.
This may be considerably less for women who
aren’t sensitive to progestogens because of their low estrogen levels, or it could be substantially extra if they’ve
excessive endogenous progestogenic ranges. Caber is the generic name for an oral birth control pill,
and can be utilized to deal with any of the aforementioned unwanted effects.
It is also a really efficient treatment for extreme bleeding during
being pregnant and could be taken along side norethindrone to alleviate
bleeding at the same time.
I loved as much as you’ll receive carried out right here. The sketch is attractive, your authored material stylish. nonetheless, you command get got an edginess over that you wish be delivering the following. unwell unquestionably come further formerly again since exactly the same nearly very often inside case you shield this hike.
I’m extremely inspired with your writing talents and also with the layout to your blog. Is that this a paid topic or did you modify it yourself? Either way keep up the excellent quality writing, it?s uncommon to look a great blog like this one nowadays..
I have observed that in video cameras, special devices help to {focus|concentrate|maintain focus|target|a**** automatically. The sensors associated with some camcorders change in in the area of contrast, while others utilize a beam involving infra-red (IR) light, specially in low lighting. Higher specification cameras from time to time use a mix of both programs and could have Face Priority AF where the photographic camera can ‘See’ a face as you concentrate only in that. Many thanks for sharing your ideas on this site.
okmark your blog and check again here regularly. I’m quite sure I will learn a lot of new stuff right here! Good luck for the next!
Today, taking into consideration the fast way of living that everyone is having, credit cards get this amazing demand throughout the economy. Persons out of every area of life are using credit card and people who not using the credit cards have lined up to apply for one. Thanks for discussing your ideas in credit cards.
Definitely believe that which you stated. Your favorite justification seemed to be on the net the easiest factor to understand of. I say to you, I definitely get annoyed even as folks think about worries that they plainly don’t understand about. You managed to hit the nail upon the top and also defined out the entire thing with no need side effect , people can take a signal. Will likely be back to get more. Thanks
70918248
References:
anabolic steroids stacks for sale (allaboutawnings.Co.uk)
My programmer is trying to convince me to move to .net from PHP. I have always disliked the idea because of the expenses. But he’s tryiong none the less. I’ve been using Movable-type on several websites for about a year and am anxious about switching to another platform. I have heard good things about blogengine.net. Is there a way I can transfer all my wordpress content into it? Any kind of help would be greatly appreciated!
I have observed that over the course of developing a relationship with real estate entrepreneurs, you’ll be able to come to understand that, in most real estate financial transaction, a payment is paid. Ultimately, FSBO sellers will not “save” the payment. Rather, they try to earn the commission by simply doing the agent’s occupation. In completing this task, they spend their money in addition to time to complete, as best they’re able to, the assignments of an realtor. Those jobs include revealing the home by way of marketing, delivering the home to buyers, creating a sense of buyer emergency in order to prompt an offer, making arrangement for home inspections, dealing with qualification checks with the loan company, supervising maintenance tasks, and facilitating the closing.
you might have a terrific weblog here! would you like to make some invite posts on my blog?
It?s actually a cool and helpful piece of information. I am glad that you shared this helpful info with us. Please keep us informed like this. Thanks for sharing.
Heya i am for the primary time here. I found this board and I in finding It really useful & it helped me out much. I am hoping to offer something back and aid others like you aided me.
I think one of your commercials caused my web browser to resize, you may well want to put that on your blacklist.
70918248
References:
how to Get anabolic steroids – wilsonrivercustomrods.com,
Thank you, I have been searching for information about this subject matter for ages and yours is the best I have located so far.
70918248
References:
Steroid Sustanon 250 [Claudiomaia.Com]
I loved as much as you will receive carried out right here. The sketch is tasteful, your authored subject matter stylish. nonetheless, you command get bought an edginess over that you wish be delivering the following. unwell unquestionably come more formerly again as exactly the same nearly very often inside case you shield this hike.
70918248
References:
none – benzincafe.com.au –
you are really a excellent webmaster. The web site loading speed is amazing. It seems that you’re doing any unique trick. Moreover, The contents are masterpiece. you have performed a excellent activity in this topic!
Thanks for these guidelines. One thing I should also believe is the fact credit cards giving a 0 rate of interest often attract consumers together with zero interest rate, instant endorsement and easy on the web balance transfers, nevertheless beware of the most recognized factor that will certainly void your own 0 easy street annual percentage rate and also throw you out into the terrible house rapid.
check out https://s3.amazonaws.com/photovoltaik-buchloe/unlocking-the-secrets-of-photovoltaik-buchloe-a-complete-guide.html
According to my observation, after a in foreclosure process home is bought at a bidding, it is common for your borrower in order to still have a remaining unpaid debt on the mortgage loan. There are many loan merchants who attempt to have all rates and liens paid by the future buyer. On the other hand, depending on specified programs, legislation, and state laws there may be several loans which aren’t easily resolved through the shift of personal loans. Therefore, the duty still falls on the consumer that has got his or her property in foreclosure. Many thanks for sharing your ideas on this site.
I have seen plenty of useful factors on your web site about pcs. However, I’ve the viewpoint that netbooks are still less than powerful more than enough to be a option if you normally do jobs that require loads of power, such as video touch-ups. But for website surfing, microsoft word processing, and quite a few other common computer work they are okay, provided you can’t mind the screen size. Many thanks for sharing your opinions.
I loved up to you’ll obtain carried out right here. The comic strip is attractive, your authored material stylish. nevertheless, you command get got an impatience over that you wish be handing over the following. sick surely come more in the past again since exactly the similar just about a lot ceaselessly inside of case you protect this increase.
awesome
Thanks for revealing your ideas. The one thing is that learners have an option between fed student loan along with a private student loan where it can be easier to choose student loan debt consolidation reduction than through the federal education loan.
Hi, i think that i saw you visited my web site thus i came to ?return the favor?.I’m attempting to find things to enhance my web site!I suppose its ok to use some of your ideas!!
Thanks for the advice on credit repair on your blog. Some tips i would tell people should be to give up the actual mentality they will buy now and pay out later. Being a society all of us tend to repeat this for many factors. This includes holidays, furniture, and items we want. However, it is advisable to separate one’s wants from the needs. When you are working to boost your credit score actually you need some sacrifices. For example you’ll be able to shop online to save cash or you can click on second hand stores instead of highly-priced department stores for clothing.
nenarazili jste někdy na problémy s plagorismem nebo porušováním autorských práv? Moje webové stránky mají spoustu unikátního obsahu, který jsem vytvořil.
Do you mind if I quote a couple of your articles as long as I provide credit and sources back to your blog? My blog is in the exact same niche as yours and my visitors would really benefit from a lot of the information you provide here. Please let me know if this ok with you. Appreciate it!
Hello there! Would you mind if I share your blog with my zynga group? There’s a lot of folks that I think would really appreciate your content. Please let me know. Cheers
One thing is that when you are searching for a student loan you may find that you will want a co-signer. There are many circumstances where this is true because you will find that you do not possess a past credit standing so the financial institution will require that you’ve got someone cosign the money for you. Thanks for your post.
check out https://s3.ap-south-1.amazonaws.com/restumpingbendigo/ultimate-guide-to-restumping-in-bendigo-everything-you-need-to-know.html
check out https://storage.googleapis.com/restumpingbendigo/understanding-the-risk-importance-of-restumping-in-bendigo-homes.html
check out https://storage.googleapis.com/restumpingbendigo/restumping-revolution-modernizing-bendigo-properties.html
You need to read https://storage.googleapis.com/concretedrivewaysinmelbourne/5-easy-steps-to-upgrade-your-concrete-driveway-in-melbourne.html
Thanks for your article. It is rather unfortunate that over the last several years, the travel industry has had to deal with terrorism, SARS, tsunamis, flu virus, swine flu, plus the first ever entire global economic depression. Through it all the industry has proven to be strong, resilient plus dynamic, discovering new methods to deal with hardship. There are continually fresh complications and possibilities to which the field must just as before adapt and answer.
Just read the article and I have to say—really well done. It was super informative, easy to follow, and actually learned a a few things new things. Definitely worth the read!
One other important aspect is that if you are a senior citizen, travel insurance regarding pensioners is something you need to really consider. The mature you are, greater at risk you happen to be for having something poor happen to you while in foreign countries. If you are not really covered by a few comprehensive insurance plan, you could have a number of serious issues. Thanks for discussing your advice on this website.
Thanks for these guidelines. One thing I should also believe is that credit cards giving a 0 monthly interest often appeal to consumers with zero interest rate, instant authorization and easy on-line balance transfers, however beware of the main factor that can void that 0 easy road annual percentage rate plus throw anybody out into the very poor house quick.